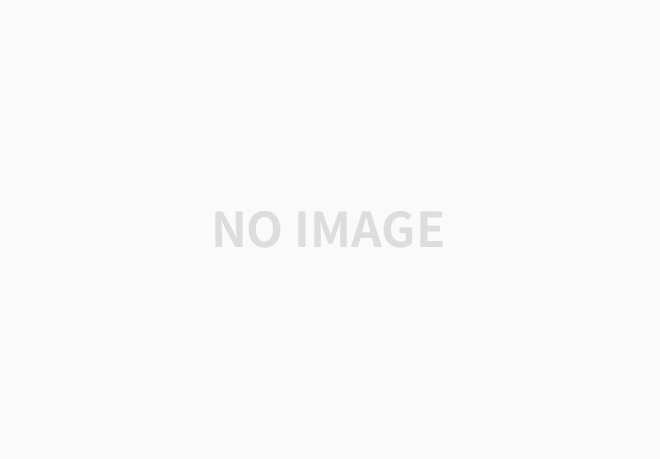
주사위 게임
아주 간단하게 주사위를 굴려서 높은 숫자가 나온 사람이 이기는 게임
처음이니 봇이랑 자신만 해서 대결을 해보자
아래는 저번 코드이다.
import asyncio, discord
from discord.ext import commands
bot = commands.Bot(command_prefix="$")
@bot.event
async def on_ready():
print("We have loggedd in as {0.user}".format(bot))
@bot.command()
async def hello(ctx):
await ctx.send("hello")
@bot.event
async def on_command_error(ctx, error):
if isinstance(error, commands.CommandNotFound):
await ctx.send("명령어를 찾지 못했습니다")
bot.run('your token here')
사실 그냥 랜덤값 뽑고 비교한 다음 출력만 하면 되기 때문에 코딩을 할 줄 안다면 별거 없다.
import asyncio, discord
import random
from discord.ext import commands
bot = commands.Bot(command_prefix="$")
@bot.event
async def on_ready():
print("We have loggedd in as {0.user}".format(bot))
@bot.command()
async def hello(ctx):
await ctx.send("hello")
@bot.command()
async def 주사위(ctx):
await ctx.send("주사위를 굴립니다.")
a = random.randrange(1,7)
b = random.randrange(1,7)
if a > b:
await ctx.send("패배")
await ctx.send("봇의 숫자: " + str(a) + " 당신의 숫자: " + str(b))
elif a == b:
await ctx.send("무승부")
await ctx.send("봇의 숫자: " + str(a) + " 당신의 숫자: " + str(b))
elif a < b:
await ctx.send("승리")
await ctx.send("봇의 숫자: " + str(a) + " 당신의 숫자: " + str(b))
@bot.event
async def on_command_error(ctx, error):
if isinstance(error, commands.CommandNotFound):
await ctx.send("명령어를 찾지 못했습니다")
bot.run("your token here")
실행결과는 아래와 같다
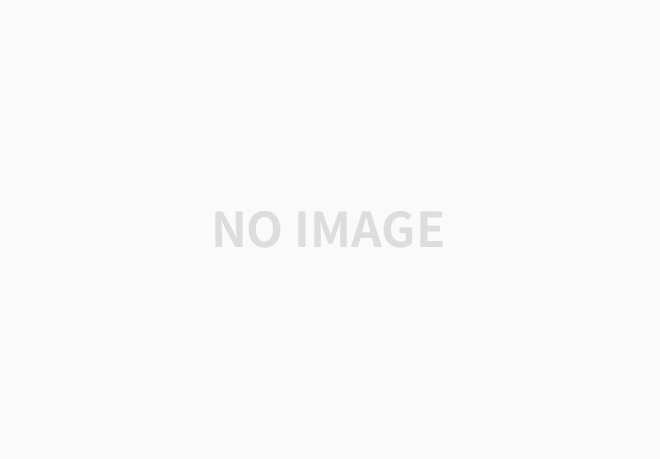
너무 재미없어 보인다.
보통 봇들은 이모티콘도 사용하고 그러던데...
코드 분리
일단 코드가 너무 길어지니 메인 파일(run.py)과 주사위 게임 파일(dice.py)로 나누었다.
#main.py
import asyncio, discord
from dice import *
from discord.ext import commands
bot = commands.Bot(command_prefix="$")
@bot.event
async def on_ready():
print("We have loggedd in as {0.user}".format(bot))
@bot.command()
async def hello(ctx):
await ctx.send("hello")
@bot.command()
async def 주사위(ctx):
await ctx.send("주사위를 굴립니다.")
await ctx.send(dice())
@bot.event
async def on_command_error(ctx, error):
if isinstance(error, commands.CommandNotFound):
await ctx.send("명령어를 찾지 못했습니다")
bot.run("Nzk0NDMzMTgzNjYzMTI4NjE2.X-6vjg.40Wtu0bCgSRo_aE3Ojtj2vm8peI")
#dice.py
import random
def dice():
a = random.randrange(1,7)
b = random.randrange(1,7)
if a > b:
return "패배\n봇의 숫자: " + str(a) + " 당신의 숫자: " + str(b)
elif a == b:
return "무승부\n봇의 숫자: " + str(a) + " 당신의 숫자: " + str(b)
elif a < b:
return "승리\n봇의 숫자: " + str(a) + " 당신의 숫자: " + str(b)
별로 좋은 코드는 아니다.
이제 결과를 예쁘게 꾸며보자
Embed 사용하기
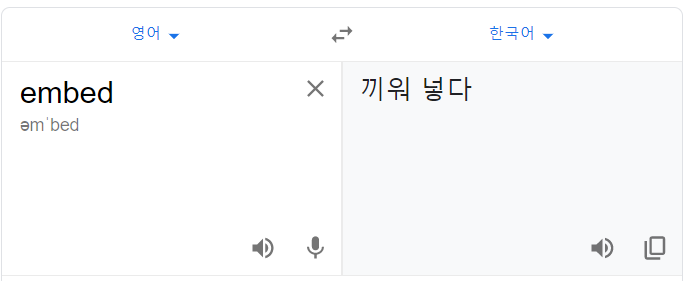
embed는 끼워넣다라는 뜻으로 웹을 좀 배웠다면 친숙한 단어일 것이다.
디스코드에서의 embed는 아래와 같이 표시된다.
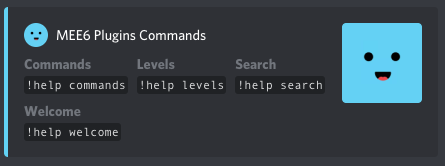
주사위를 굴리면 숫자에 따라 이모티콘과 함께 승패에 따른 색깔도 바꾸어 출력해보자.
사용할 이모지는 :game_die:이다. 아쉽게도 기본 이모지중에는 1~6 주사위가 없는 것 같다.

일반 이모티콘으로는 1~6 주사위가 있지만 잘 보이지 않는다.

embed는 다음과 같이 사용한다.
embed = discord.Embed(title = "제목", description = "설명", color = [Hex])
embed.add_field(name = "필드이름", value = "필드설명", inline = True)
embed.set_footer(text = "footer")
await ctx.send(embed = embed)
inline은 True일때는 옆으로 배열하고 False일때는 필드마다 줄바꿈을 한다.
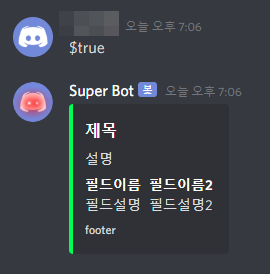
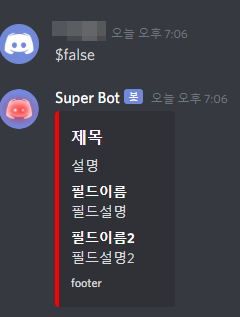
응용하여 주사위 게임 결과를 꾸며보자
#run.py
import asyncio, discord
from dice import *
from discord.ext import commands
bot = commands.Bot(command_prefix="$")
@bot.event
async def on_ready():
print("We have loggedd in as {0.user}".format(bot))
@bot.command()
async def hello(ctx):
await ctx.send("hello")
@bot.command()
async def 주사위(ctx):
result, _color, bot, user = dice()
embed = discord.Embed(title = "주사위 게임 결과", description = None, color = _color)
embed.add_field(name = "Super Bot의 숫자", value = ":game_die: " + bot, inline = True)
embed.add_field(name = ctx.author.name+"의 숫자", value = ":game_die: " + user, inline = True)
embed.set_footer(text="결과: " + result)
await ctx.send(embed=embed)
@bot.event
async def on_command_error(ctx, error):
if isinstance(error, commands.CommandNotFound):
await ctx.send("명령어를 찾지 못했습니다")
bot.run("your token here")
#dice.py
import random
def dice():
a = random.randrange(1,7)
b = random.randrange(1,7)
if a > b:
return "패배", 0xFF0000, str(a), str(b)
elif a == b:
return "무승부", 0xFAFA00, str(a), str(b)
elif a < b:
return "승리", 0x00ff56, str(a), str(b)
dice.py에서 결과, 표시색상, 봇의 주사위 결과, 유저의 주사위 결과를 반환하고
run.py에서 각 반환값을 embed에 넣는다.
결과는 아래와 같다.
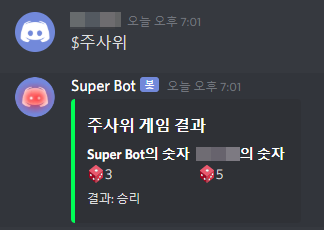
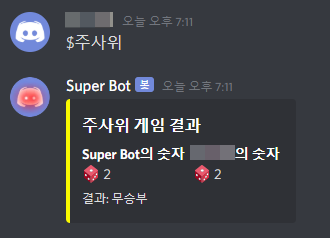
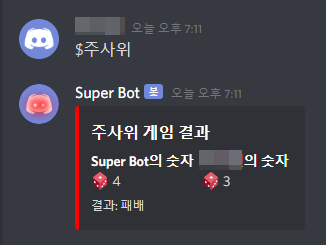
그냥 텍스트만 나왔을 때보다 훨씬 예뻐 보인다.
다음엔 음악 봇을 만들어보겠다.
'프로젝트 > 디스코드 봇' 카테고리의 다른 글
디스코드 봇 만들기#5 - 음악재생 (2) | 2021.02.05 |
---|---|
디스코드 봇 만들기#4 - 음성채널 (7) | 2021.01.29 |
디스코드 봇 만들기#2 - 간단한 명령어 (10) | 2021.01.15 |
디스코드 봇 만들기#1 - 개발 준비하기 (3) | 2021.01.08 |
디스코드 봇 만들기#0 - 프로젝트 개요 (0) | 2021.01.01 |